INSTALL Selenium module for Python
## Assuming that you already have Python 3 installed
C:\windows\system32> pip install selenium
|
DOWNLOAD Webdrivers
## Download the webdriver for the required browsers to automate
## Unzip these drivers and place the .exe file into a folder that is Windows PATH for all users
e.g. if C:\Python3 is already in PATH, copy the webdriver .exe into c:\python3
e.g. if not, put into a folder such as c:\webdriver and then open a command prompt as administrator and
C:\windows\system32> SETX /M PATH "%PATH%;C:\webdriver"
|
TEST RUN
## create a test python script browser.py as follows and execute
c:\scripts\browse.py |
from selenium import webdriver
from selenium.webdriver.common.keys import Keys
driver = webdriver.Chrome()
driver.get("http://www.python.org")
assert "Python" in driver.title
elem = driver.find_element_by_name("q")
elem.clear()
elem.send_keys("pycon")
elem.send_keys(Keys.RETURN)
|
## It should launch Chrome browser and then load the URL and enter "pycon" into the search box and press Return
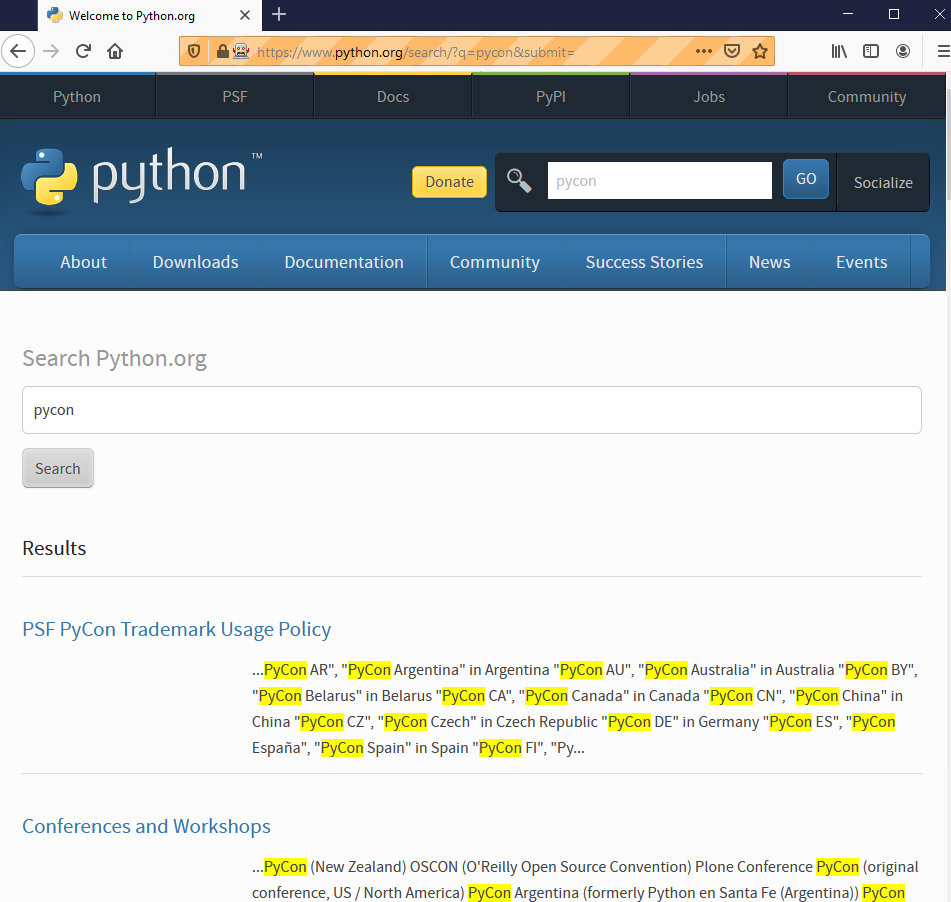
## to close the Chrome browser automatically add to the bottom of the script
driver.close()
|
DISCOVERING THE Selenium API
## launch Python intepreter and then
>>> import selenium
>>> help('selenium.webdriver.Firefox')
|
REFERENCES
|